- Published on
How To Make A (very) simple modal With Tailwind CSS In 5 Easy Steps
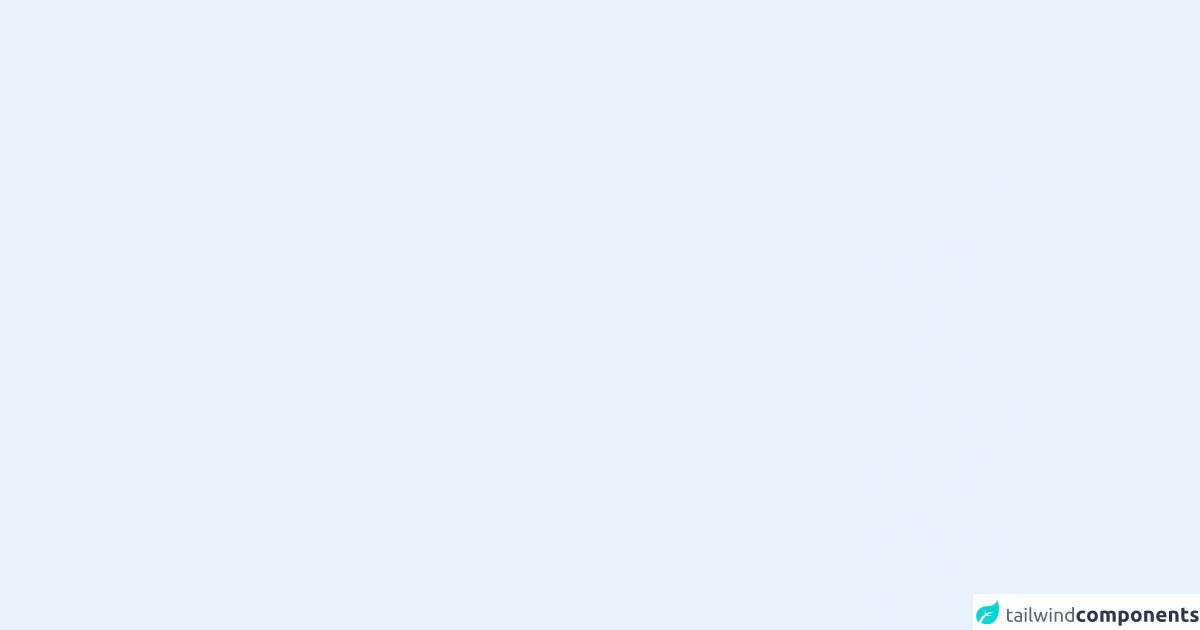
- What is Tailwind CSS?
- The description of (very) simple modal ui component
- Why use Tailwind CSS to create a (very) simple modal ui component?
- The preview of (very) simple modal ui component.
- The source code of (very) simple modal ui component.
- How to create a (very) simple modal with Tailwind CSS?
- Step 1: Set up the HTML structure
- Step 2: Set up the CSS styles
- Step 3: Add JavaScript to show and hide the modal
- Step 4: Test the modal
- Step 5: Customize the modal
- Conclusion
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that provides a set of pre-defined classes to create responsive and customizable user interfaces. It allows developers to quickly and easily create complex layouts and designs without writing custom CSS code.
The description of (very) simple modal ui component
A modal is a user interface component that displays content on top of the current page, blocking out the rest of the content. It is commonly used to display important information, confirmations, or to collect user input.
In this tutorial, we will create a (very) simple modal using Tailwind CSS. This modal will consist of a button that, when clicked, will display a modal with some text and a close button.
Why use Tailwind CSS to create a (very) simple modal ui component?
Tailwind CSS provides a set of pre-defined classes that can be used to create complex user interfaces quickly and easily. It also provides a responsive design system that makes it easy to create layouts that work on different screen sizes.
By using Tailwind CSS to create a (very) simple modal, we can save time and effort by not having to write custom CSS code. We can also take advantage of Tailwind's responsive design system to make our modal work on different screen sizes.
The preview of (very) simple modal ui component.
To create a (very) simple modal using Tailwind CSS, we will need to use some HTML and CSS code. The modal will consist of a button that, when clicked, will display a modal with some text and a close button.
Free download of the (very) simple modal's source code
The source code of (very) simple modal ui component.
To create our (very) simple modal, we will need to use some HTML and CSS code. The HTML code will define the structure of our modal, and the CSS code will style it.
<!--Open modal button-->
<div>
<button id="buttonmodal" class="focus:outline-none p-2 bg-blue-600 text-white bg-opacity-75 rounded-lg ring-4 ring-indigo-300" type="button">Open modal</button>
</div>
<div id="modal"
class="fixed top-0 left-0 w-screen h-screen flex items-center justify-center bg-blue-500 bg-opacity-50 transform scale-0 transition-transform duration-300">
<!-- Modal content -->
<div class="bg-white w-1/2 h-1/2 p-12">
<!--Close modal button-->
<button id="closebutton" type="button" class="focus:outline-none">
<!-- Hero icon - close button -->
<svg xmlns="http://www.w3.org/2000/svg" class="h-6 w-6" fill="none" viewBox="0 0 24 24"
stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2"
d="M10 14l2-2m0 0l2-2m-2 2l-2-2m2 2l2 2m7-2a9 9 0 11-18 0 9 9 0 0118 0z" />
</svg>
</button>
<!-- Test content -->
<p>
Lorem, ipsum dolor sit amet consectetur adipisicing elit.
Minus placeat maiores repudiandae, error perferendis inventore
aspernatur voluptatum omnis sint debitis!
</p>
</div>
</div>
<script>
const button = document.getElementById('buttonmodal')
const closebutton = document.getElementById('closebutton')
const modal = document.getElementById('modal')
button.addEventListener('click',()=>modal.classList.add('scale-100'))
closebutton.addEventListener('click',()=>modal.classList.remove('scale-100'))
</script>
How to create a (very) simple modal with Tailwind CSS?
Now that we have an idea of what we want to create, let's get started with the tutorial. Follow these five easy steps to create a (very) simple modal with Tailwind CSS.
Step 1: Set up the HTML structure
The first step is to set up the HTML structure for our modal. We will create a button that, when clicked, will display our modal.
<button class="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded">
Open Modal
</button>
<div class="modal">
<div class="modal-content">
<span class="close">×</span>
<p>Some text in the modal.</p>
</div>
</div>
In this code, we have created a button with the class bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded
. This class will style our button with a blue background color, white text, and rounded corners.
We have also created a div
element with the class modal
that will contain our modal content. Inside this div
, we have created another div
element with the class modal-content
that will contain the text and close button.
Step 2: Set up the CSS styles
The next step is to set up the CSS styles for our modal. We will use Tailwind CSS classes to style our modal.
/* Modal styles */
.modal {
display: none;
position: fixed;
z-index: 1;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgba(0,0,0,0.4);
}
.modal-content {
background-color: #fefefe;
margin: 15% auto;
padding: 20px;
border: 1px solid #888;
width: 80%;
}
.close {
color: #aaa;
float: right;
font-size: 28px;
font-weight: bold;
}
.close:hover,
.close:focus {
color: black;
text-decoration: none;
cursor: pointer;
}
In this code, we have defined the styles for our modal. The .modal
class sets the modal to be hidden by default and positions it at the top left corner of the screen. The .modal-content
class sets the background color, margin, padding, and border of the modal content. The .close
class sets the styles for the close button.
Step 3: Add JavaScript to show and hide the modal
The next step is to add JavaScript code to show and hide the modal when the button is clicked.
// Get the modal
var modal = document.querySelector('.modal');
// Get the button that opens the modal
var btn = document.querySelector('button');
// Get the <span> element that closes the modal
var span = document.querySelector('.close');
// When the user clicks the button, open the modal
btn.onclick = function() {
modal.style.display = "block";
}
// When the user clicks on <span> (x), close the modal
span.onclick = function() {
modal.style.display = "none";
}
// When the user clicks anywhere outside of the modal, close it
window.onclick = function(event) {
if (event.target == modal) {
modal.style.display = "none";
}
}
In this code, we have defined some JavaScript code to show and hide the modal. When the user clicks the button, we set the display
property of the modal to block
to show it. When the user clicks the close button or clicks outside the modal, we set the display
property of the modal to none
to hide it.
Step 4: Test the modal
The next step is to test our modal to make sure it works as expected. Open the HTML file in your web browser and click the button. The modal should appear with some text and a close button. Click the close button or click outside the modal to hide it.
Step 5: Customize the modal
The final step is to customize the modal to fit your needs. You can change the text, styles, and behavior of the modal to match your requirements.
Conclusion
In this tutorial, we have learned how to create a (very) simple modal using Tailwind CSS. We have used HTML, CSS, and JavaScript code to create a modal that displays some text and a close button. By using Tailwind CSS, we have saved time and effort by not having to write custom CSS code. We have also taken advantage of Tailwind's responsive design system to make our modal work on different screen sizes.