- Published on
How to Build A Pure CSS Dropdown Menu With Tailwind CSS?
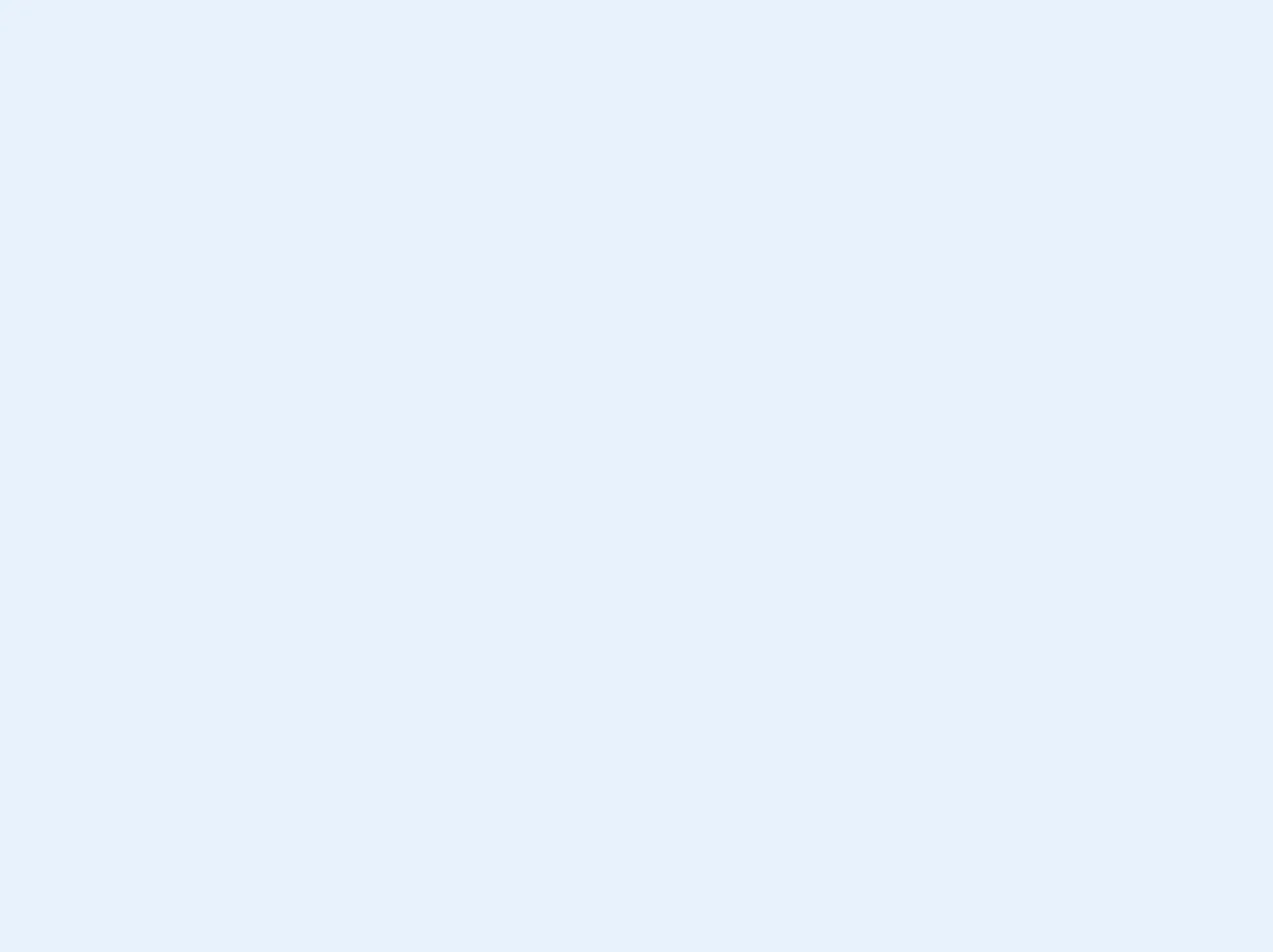
- What is Tailwind CSS?
- The description of Pure CSS Dropdown Menu ui component
- Why use Tailwind CSS to create a Pure CSS Dropdown Menu ui component?
- The preview of Pure CSS Dropdown Menu ui component.
- The source code of Pure CSS Dropdown Menu ui component.
- How to create a Pure CSS Dropdown Menu with Tailwind CSS?
- Conclusion
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that allows you to easily create custom designs without writing any CSS code. It provides a set of pre-defined classes that can be used to style HTML elements. Tailwind CSS is gaining popularity among developers due to its simplicity and flexibility.
The description of Pure CSS Dropdown Menu ui component
A dropdown menu is a common UI component used in web applications. It allows users to select an option from a list of options. A pure CSS dropdown menu is a menu that is created using only CSS code, without any JavaScript code. In this article, we will learn how to create a pure CSS dropdown menu using Tailwind CSS.
Why use Tailwind CSS to create a Pure CSS Dropdown Menu ui component?
Tailwind CSS provides a set of pre-defined classes that can be used to style HTML elements. This makes it easy to create custom designs without writing any CSS code. Tailwind CSS also provides a responsive design system that allows you to create designs that work on different screen sizes. Using Tailwind CSS to create a pure CSS dropdown menu is a great way to create a custom UI component that is easy to maintain and customize.
The preview of Pure CSS Dropdown Menu ui component.
To create a pure CSS dropdown menu, we will use Tailwind CSS classes to style the HTML elements. The menu will have a simple design with a dropdown button and a list of options.
Free download of the Pure CSS Dropdown Menu's source code
The source code of Pure CSS Dropdown Menu ui component.
To create a pure CSS dropdown menu, we will use HTML and CSS code. The HTML code will define the structure of the menu, while the CSS code will style the menu using Tailwind CSS classes.
<style>
#sortbox:checked ~ #sortboxmenu {
opacity: 1;
}
</style>
<div class="relative">
<input type="checkbox" id="sortbox" class="hidden absolute">
<label for="sortbox" class="flex items-center space-x-1 cursor-pointer">
<span class="text-lg">Sort By</span>
<svg class="h-4 w-4" xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M19 9l-7 7-7-7" />
</svg>
</label>
<div id="sortboxmenu" class="absolute mt-1 right-1 top-full min-w-max shadow rounded opacity-0 bg-gray-300 border border-gray-400 transition delay-75 ease-in-out z-10">
<ul class="block text-right text-gray-900">
<li><a href="#" class="block px-3 py-2 hover:bg-gray-200">Featured</a></li>
<li><a href="#" class="block px-3 py-2 hover:bg-gray-200">Newest</a></li>
<li><a href="#" class="block px-3 py-2 hover:bg-gray-200">Price: Low to High</a></li>
<li><a href="#" class="block px-3 py-2 hover:bg-gray-200">Price: High to Low</a></li>
</ul>
</div>
</div>
How to create a Pure CSS Dropdown Menu with Tailwind CSS?
To create a pure CSS dropdown menu with Tailwind CSS, follow these steps:
- Create the HTML structure for the menu.
<div class="relative inline-block text-left">
<button type="button" class="inline-flex justify-center w-full rounded-md border border-gray-300 shadow-sm px-4 py-2 bg-white text-sm font-medium text-gray-700 hover:bg-gray-50 focus:outline-none focus:ring-2 focus:ring-offset-2 focus:ring-indigo-500" id="menu-button" aria-expanded="false" aria-haspopup="true">
Dropdown
<!-- Heroicon name: chevron-down -->
<svg class="-mr-1 ml-2 h-5 w-5" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 20 20" fill="currentColor" aria-hidden="true">
<path fill-rule="evenodd" d="M5.293 7.293a1 1 0 011.414 0L10 10.586l3.293-3.293a1 1 0 011.414 1.414l-4 4a1 1 0 01-1.414 0l-4-4a1 1 0 010-1.414z" clip-rule="evenodd" />
</svg>
</button>
<div class="origin-top-right absolute right-0 mt-2 w-56 rounded-md shadow-lg bg-white ring-1 ring-black ring-opacity-5 focus:outline-none" role="menu" aria-orientation="vertical" aria-labelledby="menu-button" tabindex="-1">
<div class="py-1" role="none">
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100 hover:text-gray-900" role="menuitem" tabindex="-1" id="menu-item-0">Account settings</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100 hover:text-gray-900" role="menuitem" tabindex="-1" id="menu-item-1">Support</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100 hover:text-gray-900" role="menuitem" tabindex="-1" id="menu-item-2">License</a>
<form method="POST" action="#" role="none">
<button type="submit" class="block w-full text-left px-4 py-2 text-sm text-gray-700 hover:bg-gray-100 hover:text-gray-900" role="menuitem" tabindex="-1" id="menu-item-3">
Sign out
</button>
</form>
</div>
</div>
</div>
- Style the menu using Tailwind CSS classes.
<style>
.dropdown-menu {
@apply relative inline-block text-left;
}
.dropdown-menu button {
@apply inline-flex justify-center w-full rounded-md border border-gray-300 shadow-sm px-4 py-2 bg-white text-sm font-medium text-gray-700 hover:bg-gray-50 focus:outline-none focus:ring-2 focus:ring-offset-2 focus:ring-indigo-500;
}
.dropdown-menu button svg {
@apply -mr-1 ml-2 h-5 w-5;
}
.dropdown-menu .menu {
@apply origin-top-right absolute right-0 mt-2 w-56 rounded-md shadow-lg bg-white ring-1 ring-black ring-opacity-5 focus:outline-none;
}
.dropdown-menu .menu a {
@apply block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100 hover:text-gray-900;
}
.dropdown-menu .menu form button {
@apply block w-full text-left px-4 py-2 text-sm text-gray-700 hover:bg-gray-100 hover:text-gray-900;
}
</style>
- Add JavaScript code to handle the dropdown menu.
<script>
const button = document.getElementById('menu-button');
const menu = document.querySelector('.menu');
button.addEventListener('click', () => {
const expanded = button.getAttribute('aria-expanded') === 'true' || false;
button.setAttribute('aria-expanded', !expanded);
menu.classList.toggle('hidden');
});
</script>
Conclusion
In this article, we learned how to create a pure CSS dropdown menu using Tailwind CSS. We used Tailwind CSS classes to style the HTML elements and added JavaScript code to handle the dropdown menu. Creating a pure CSS dropdown menu with Tailwind CSS is a great way to create a custom UI component that is easy to maintain and customize.