- Published on
Learn How To Make A Range Slider With Tailwind CSS from the Pros
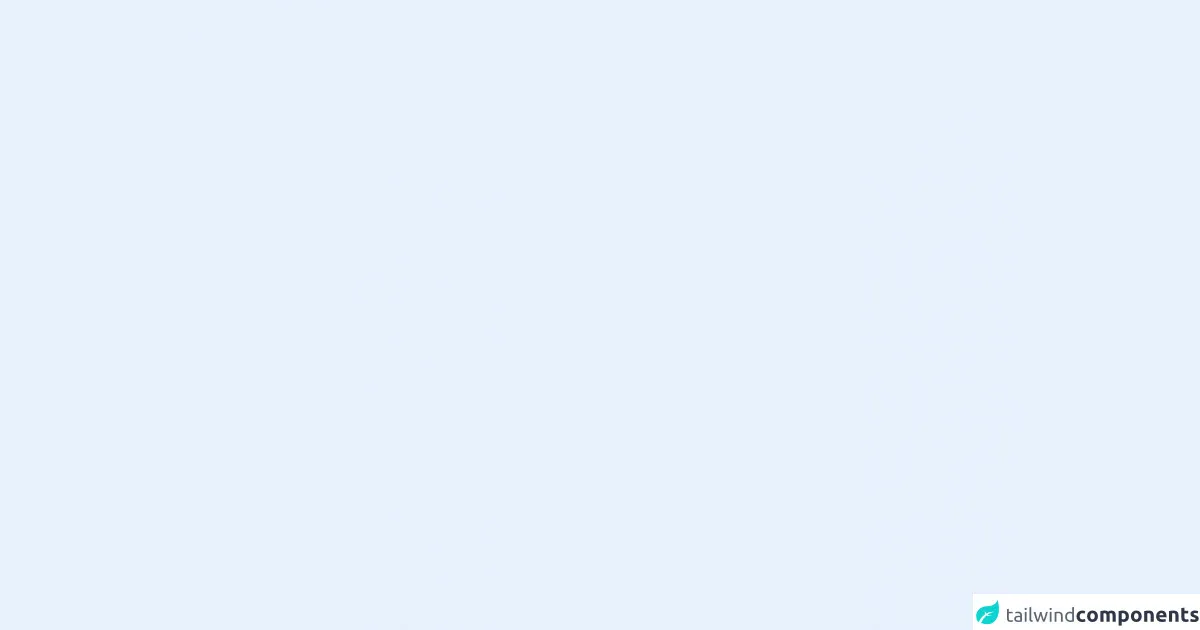
- What is Tailwind CSS?
- The description of Range Slider ui component
- Why use Tailwind CSS to create a Range Slider ui component?
- The preview of Range Slider ui component
- The source code of Range Slider ui component
- How to create a Range Slider with Tailwind CSS?
- Conclusion
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that helps you quickly build custom designs. It provides a set of pre-defined classes that you can use to style your HTML elements. Instead of writing CSS from scratch, you can use Tailwind's classes to create a responsive and consistent design.
The description of Range Slider ui component
A range slider is a UI component that allows users to select a range of values by dragging a slider handle. It is commonly used in forms, filters, and other interactive interfaces. The range slider consists of a track, two handles, and a fill that shows the selected range.
Why use Tailwind CSS to create a Range Slider ui component?
Tailwind CSS provides a set of pre-defined classes that you can use to create a range slider quickly. You don't need to write custom CSS from scratch, which saves you time and effort. Additionally, Tailwind's classes are responsive, which means that your range slider will automatically adjust to different screen sizes.
The preview of Range Slider ui component
To create a range slider with Tailwind CSS, you can use the range
class. This class applies the default styles for a range slider, including the track, handles, and fill. You can customize the appearance of the range slider by adding additional classes or modifying the existing ones.
Free download of the Range Slider's source code
The source code of Range Slider ui component
To create a range slider with Tailwind CSS, you can use the following HTML code:
<div class="range">
<input type="range" class="range-slider" min="0" max="100" value="50">
</div>
This code creates a range slider with a minimum value of 0, a maximum value of 100, and an initial value of 50. The range
class applies the default styles for the range slider, while the range-slider
class sets the appearance of the slider handle.
<script src="https://cdn.jsdelivr.net/gh/alpinejs/[email protected]/dist/alpine.min.js" defer></script>
<div class="flex flex-col items-center" x-data="rangeSlider(18, 80, 25, 55)">
<input class="absolute opacity-0 pointer-events-none"
type="range" name="age_min" min="18" max="80" :value="min">
<input class="absolute opacity-0 pointer-events-none"
type="range" name="age_max" min="18" max="80" :value="max">
<span x-text="`${min} to ${max}`"></span>
<div class="flex items-center relative rounded bg-gray-300 w-40 h-2 mt-4"
x-ref="sliderEl"
@mouseup.window="dragLeft = dragRight = false"
@mousemove.window="handleThumbMouseMove($event)"
style="user-select: none"
>
<div class="absolute h-2 bg-blue-400"
:style="`left: ${(min - rangeMin) * 100 / range}%; right: ${100 - (max - rangeMin) * 100 / range}%`"></div>
<div class="w-4 h-4 -ml-2 rounded-full bg-blue-500 absolute cursor-pointer"
@mousedown="dragLeft = true"
:style="`left: ${(min - rangeMin) * 100 / range}%`"
x-ref="minThumb"></div>
<div class="w-4 h-4 -ml-2 rounded-full bg-blue-500 absolute cursor-pointer"
@mousedown="dragRight = true"
:style="`left: ${(max - rangeMin) * 100 / range}%`"
x-ref="maxThumb"></div>
</div>
</div>
<script>
function rangeSlider(rangeMin, rangeMax, min, max) {
return {
range: rangeMax - rangeMin,
rangeMin,
rangeMax,
min,
max,
dragLeft: false,
dragRight: false,
handleThumbMouseMove: function(e) {
if (!this.dragLeft && !this.dragRight) return;
const thumbEl = this.dragLeft ? this.$refs.minThumb : this.$refs.maxThumb;
const sliderRect = this.$refs.sliderEl.getBoundingClientRect();
let r = (e.clientX - sliderRect.left) / sliderRect.width;
r = Math.max(0, Math.min(r, 1));
const value = Math.floor(r * this.range + this.rangeMin);
if (this.dragLeft) {
this.min = value;
this.max = Math.max(this.min, this.max);
} else {
this.max = value;
this.min = Math.min(this.min, this.max);
}
}
};
}
</script>
How to create a Range Slider with Tailwind CSS?
To create a custom range slider with Tailwind CSS, you can use the following steps:
Define the HTML structure: Create a
div
element with therange
class and aninput
element with therange-slider
class. Set themin
,max
, andvalue
attributes of the input element to define the range of values.Style the track: Use the
bg-gray-300
class to set the background color of the track. You can also use theh-1
class to set the height of the track.Style the fill: Use the
bg-blue-500
class to set the color of the fill. You can also use theh-1
class to set the height of the fill.Style the handles: Use the
w-4 h-4 rounded-full bg-white border-2 border-gray-300
class to set the appearance of the handles. You can also use thehover:bg-blue-500
class to change the color of the handle when the user hovers over it.Add interactivity: Use JavaScript to update the fill and handle positions when the user drags the handles. You can also use CSS transitions to create a smooth animation.
Here's an example of how to create a custom range slider with Tailwind CSS:
<div class="range">
<input type="range" class="range-slider" min="0" max="100" value="50">
<div class="fill bg-blue-500 h-1"></div>
<div class="handle left w-4 h-4 rounded-full bg-white border-2 border-gray-300"></div>
<div class="handle right w-4 h-4 rounded-full bg-white border-2 border-gray-300"></div>
</div>
This code creates a range slider with two handles and a blue fill. The handles have a white background and a gray border, and they change color to blue when the user hovers over them.
Conclusion
Creating a range slider with Tailwind CSS is a straightforward process that can save you time and effort. By using Tailwind's pre-defined classes, you can create a responsive and consistent design without writing custom CSS from scratch. With a little bit of customization, you can create a range slider that fits your specific needs.